Here are the steps to build the sample:
-Create a Blank Workspace called Shapes;
-Add the two projects: ShapesUi (ARX) and ShapesObj (DBX). Both with MFC extensions;

-Create a dependency from ShapesUi to ShapesObj;
-Compile de project with Build All option;
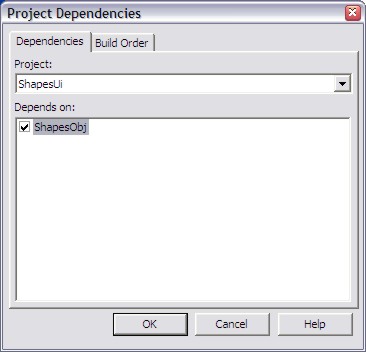
-Open Autodesk Class Explorer and right click over ShapesObj project;
-Select "Add an ObjectDBX Custom Object" option;

-Enter the Class Name as ShapeObject. DWG protocol functions are automatically enabled. Click Finish;
-Two files will be created in your ShapesObj project: ShapeObject.h and ShapeObject.cpp;
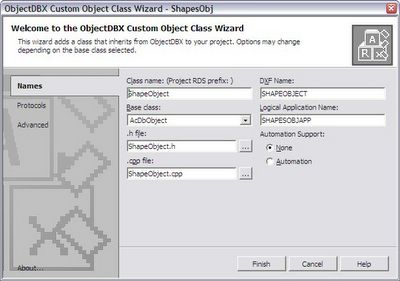
-Open Autodesk Class Explorer again and right ShapeObject class. Select Add variable...;

-Enter the variable name (I suggest you to prefix all members with m_), enable "Implement Get/Put methods" option;
-Select "Access" as protected because we will use the Get/Put functions to change variables;
-Fill the "Comment" field with a description and uncheck the "Increase version number" option. Click Finish;
-Repeat this process for each of our variables: m_d, m_tw, m_bf, m_tf and m_desig;
-Go to the header file of your custom object class and make some adjustments to group variables and functions;
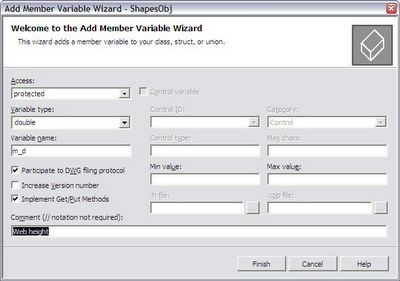
-Compile your project. You will get one error:
"cannot convert parameter 1 from 'CString *...".
This is due the problem when writing and reading CString types.
We need to make some changes into dwgInFields() and dwgOutFields() methods:
dwgOutFields:
from:
pFiler->writeItem(m_desig);
to:
pFiler->writeString(static_cast<const TCHAR*>(m_desig));
dwgInFields:
from:
pFiler->readItem(&m_desig);
to:
TCHAR* temp = NULL;
pFiler->readString(&temp);
m_desig.Format(_T("%s"),temp);
acutDelString(temp);
-Now, let's change the CString access functions:
from:
CString ShapeObject::get_m_desig(void) const
{
assertReadEnabled () ;
return (m_desig) ;
}
Acad::ErrorStatus ShapeObject::put_m_desig(CString newVal)
{
assertWriteEnabled () ;
m_desig =newVal ;
return (Acad::eOk) ;
}
to:
void ShapeObject::get_m_desig(CString& newVal) const
{
assertReadEnabled () ;
newVal.Format(_T("%s"),m_desig) ;
}
Acad::ErrorStatus ShapeObject::put_m_desig(LPCTSTR newVal)
{
assertWriteEnabled () ;
m_desig.Format(_T("%s"),newVal);
return (Acad::eOk) ;
}
This will avoid heap violation problems when passing strings from one
module to another. Remember to also change the respective function declarations.
- Open the acrxEntryPoint.cpp, inside ShapesUi project, and add
the following include:
#include "..\ShapesObj\ShapeObject.h"
- Now, we will use the Class 12 createMyObjects() and
listMyObjects() functions to deal with our objects. Add these functions to
acrxEntryPoint.cpp file, before the application class and make proper
adjustments. Remember that our application dictionary will be called "SHAPEAPP";
- Create two commands (CSHAPE and LSHAPE) and map each
one to above functions. Be creative, optimize your code as much as you can!
- Now, before create the research function we will need to add the ==
operator to our custom object:
bool operator ==(const ShapeObject& arg) {
if (&arg == this) return true;
// We will need to use a tolerance as our values are double
// Let's use 0.1
if ( (fabs(m_d - arg.m_d ) < 0.1) &&
(fabs(m_tw - arg.m_tw) < 0.1) &&
(fabs(m_bf - arg.m_bf) < 0.1) &&
(fabs(m_tf - arg.m_tf) < 0.1) )
return true;
else
return false;
}
- To create a research function use the list function as a base and try to
find an existing shape before create a new one.
- See the solved sample code to all detailed solutions.
Remember, to test the application first load the DBX module and then the ARX.
To unload, first unload the ARX module then the DBX.
Download this sample's source code here!
See you next class!
6 comments :
Thank you for this great Tutorial!
Greetings from Stollberg/Germany
finaluser
Great learning experience ..
Thanks for your outstanding effort
it definitely helps ARX hunters
Rajesh
India
Hi Fernando,
In your suggested CString workaround, I get following error in the line:
pFiler->readString(&temp);
error C2664: 'Acad::ErrorStatus AcDbDwgFiler::readString(ACHAR **)' : cannot convert parameter 1 from 'char **__w64 ' to 'ACHAR **'
Do you have any idea?
Hi TR,
The Blog examples are non-unicode. If you trying to compile these samples with ObjectARX 2007 or 2008 the code need to be adjusted to fully support UNICODE.
In this example, you need to:
- change from char to TCHAR;
- Replace your strings between "" by _T("");
These modifications will solve your compilation error.
Regards,
i'am bad at Tech English.So i find it difficult to understand your walkthrough.Would you mind reupload your sample code.I prefer studying with it.Thanks for your help
Thaks again
Post a Comment