Our second Lab will cover MFC contents and some interaction with AutoCAD. The main idea is to keep this lab simple to consolidate MFC and user interaction knowledge.
Requirements:
* Class 1 to 10;
* AutoCAD 2004/2005/2006 or compatible vertical installed;
* Visual Studio .NET 2002 installed;
* ObjectARX Wizard installed.
Objectives:
Create a simple ObjectARX module with 1 commands: DLGCOLOR. This command will create a simple MFC dialog with a color combo box and one selection button. As the user clicks on this button it will prompt user to select one or more existing entities. After click Apply it will change the color of all selected entity.
Instructions:
- Create a project called ARXLAB2 using ObjectARX Wizard;
- Enable Using MFC option with AutoCAD MFC Extensions;
- Click on the a> icon at ARXWizard's toolbar to open command dialog;
- Right click on the above portion and select New;
- Change the global an local name for DLGCOLOR and select Modal as command mode;
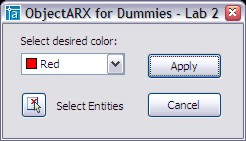
- Open the Visual Studio Editor and add a new dialog layout. Add a combo box control and a button control;
- Open the Autodesk Class Explorer, right click on the root and select Add MFC Ext. Class. Select CAcUiDialog as the base class;
- In the dialog layout, right click on the combo box and select Add Variable. Choose CAcUiTrueColorComboBox as its class and name it as m_color. Right click on the combo again and select Properties. Set Has Strings = True, Owner Draw = Fixed;
- Now, right click the button and select Add Variable. Choose CAcUiSelectButton as its class and name it as m_select. Right click once more, select Properties. Set Owner Draw = True.
- Add the virtual BOOL OnInitDialog() method. Inside its implementation add the following:
CAcUiDialog::OnInitDialog();
SetWindowText(_T("ObjectARX for Dummies - Lab 2"));
m_select.AutoLoad();
return TRUE;
- Map a click event to selection button. Right click on it, select Add Event Handler. Select BN_CLICKED event. Inside the event function, we will need to hide the dialog and switch to AutoCAD screen. As we have derived from CAcUiDialog it already has a couple of functions to do that. So we will need to hide the dialog, select the desired entities and then show the dialog back. Your function outline will be something like this:
BeginEditorCommand();
// Perform here the user interaction (allow user to select entities)
CompleteEditorCommand();
- Don't forget to switch the resources to your application before open the dialog:
CAcModuleResourceOverride res;
CDlgColor dlg;
dlg.DoModal();
Tips:
- Don't forget to call close() for all selected entities (use a Transaction if you prefer);
- Explore the code to understand what ARX Wizard has created for you;
- Pay attention to code syntax;
- Use the ObjectARX documentation when necessary;
Support:
If you have any questions please post your issue on this article to share with others.
Expected time:
- I will give you 3 days to accomplish this Lab;
- After this period, I will post my suggested solution for this.
21 comments :
Hi,
Is there a chance that you could elaborate a litte more into the steps...
For example, ...add a new layout dialog...
Well, since I do not have an idea [and I know I read the pre-requisites], is this done by right-click on the name of the project ARXLAB2 and selecting: Add -> Add resource -> Dialog -> New
That, provides the dialog form with two buttons: OK & Cancel
And the default name for the dialog: IDD_DIALOG1
Then I add the MFC Ext. Class, but what name goes for the "Class name:"
I select the CAcUiDialog, etc.
But for example if I provide the name DIALOG1 to the ext. class, if I try to follow the step: Set Has Strings = True ...
That property is not accesible as most of the other properties that are in black.
Thank you so much for all your help.
Luis.
I see why..... I must changed first Owner Draw = Fixed, and then back to Has Strings = Fixed
Luis.
Hi Luis,
Yes, unfortunately VStudio is not so smart as we whish.
Regarding to the way you insert the dialog into project I prefer to use Autodesk Class View, right click on tree root and choose "Add MFC Extension Class...".
The ARXWizard overrides the native VStudio wizard (if you accept the suggestion during its installation) and add more functionalities due MFC support of AcUi and AdUi classes.
Regards,
Fernando.
Hi Fernando,
Yes... on the latest attempt I did that, hmm I do not understand, where you said "Regarding to the way you insert the dialog into project I prefer to use Autodesk Class View...."
Any way I am almost there, I'm comming from to many lisp/vlisp coding and it is very hard for me.
I have done most of your samples and I having a lot of fun.... thank you so much!!!
BTW, I will post the simpleLine solution I did, base on yours, I added the ability to define the points and then draw the line, also to hear any comment you might have.
Regards,
Luis.
Hi Fernando,
When I use ssget()from MFC dialog class, when I am selecting entites on drawing if I press pan command button on toolbar, it sends *cancel* and my selection command is teminated and pan is invoked.
afterwards if I invoke ssget(), it doesn't cancel's the sset() or pan.
Is there any way to make trasperent ssget() in dialog.
(I tried Documment Lock() unlock())
Regards
Abhay Joshi
Hi Abhay,
It should work but your dialog needs to be a Modeless one and you need to hide to allow prompt/screen interaction.
Did you try to run the following sample?
http://arxdummies.blogspot.com/2005/04/lab-2-solved.html
Does it show the same problem with dynamic PAN?
Please let me know.
Regards,
Fernando.
Hi Fernando
I'm working on VS 2005 and I've done everything until the step with a button and a combobox. The problem is I can't find any place referencing to choosing class. For example as I select "add variable" to button a new window pops up and I have
access: public
variable type: CButton
control ID: IDC_BUTTON1
category: control
variable name: [EMPTY]
control type: BUTTON
and disabled ones:
max chars, min value, max value, .h file, .cpp file.
And nothing about choosing class. Is there aby other way to set this up? I did everything as you wrote.
Thanks in advance
Marek
Hi
I wrote a comment few minutes before but I just found out that everything works, sorry for the trouble.
Marek
I have the same problem as the one above that Marek said, but I cannot resolve it. I did exactly the same as you wrote, could anyone tell me the reason?
Hi,
Did you try to add the member variable through ClassView tab?
Select ClassView tab, look for your class, right click, select Add Member...
Sometimes the Variable Member Wizard generates some script errors. If this is your case, try to reinstall ARXWizard with Visual Studio closed.
Regards,
Fernando.
Hello Fernando,
I'm working my way through the labs but , as e few people before me, I got stuck on the "choose CAcUiTrueColorComboBox for class"-thing. There is nothing in the "add member variable wizard" about classes. What am i doing wrong?
Greetings,
Frank Nauwelaerts
Hi Frank,
Sometimes the Wizard present a problem with scripts. It is based on HTML.
You may try to uninstall and then reinstall the ARXWizard to see if it fix this problem.
If you are still running into problems using the "Add variable Wizard" try to do the process manually:
Map a variable as MFC CComboBox class using MFC Wizard and then replace the CComboBox class with the CAcUiTrueColorComboBox class.
Best Regards,
Hi,
Does anyone know if there is a way to change the color that is displayed for the ByLayer color in the CAcUiTrueColorComboBox. I have searched through the documentation and can only find a way to change the ByBlock color. I am trying to change the color dynamically when the user changes a layer selection. Any help would be greatly appreciated.
-Aaron
Aaron,
How are you trying to do that?
Could you provide a code snippet?
When I right click the combo box and the button, it doesn't appear the "add variable" and then I can't add. What can I do?
Hi,
Try to uninstall and then reinstall ARXWizard.
Regards
I have tested the application with commenting out this line
CAcModuleResourceOverride res;
But it goes fine and i got no error.
Documentation says that by using this statement, module resources will be selected and when this object is destroyed, default resources will be select.
But what is reason of no effect by commenting out this line. Can you further elaborate it. Thanks
Hi Fernando,
How connect database (access) from ObjectARX,
Regards,
Chatko from Yugoslavia,
Hello Chatko,
I have used native Visual Studio database support but today the best option is to use ADO.NET.
Unfortunately I don't have any samples showing that.
Regards.
Thank you for your great traning! But I have some problems with System::Windows::Forms. Could you explain me step by step how to create DLL library with forms (for VS2005 and acad 2007)and show it in ARX application? I will be very grateful
Hi there,
For .NET ObjectARX I highly recommend Kean's blog at:
http://through-the-interface.typepad.com/
Regards.
Post a Comment