Hello,
On this class we will implement the minimum ObjectARX application without use the ARXWizard. To do that we will need to create the Visual C++ project from scratch and perform some tuning on project settings.
To begin, open your Microsoft Visual C++ .NET 2002. Open File menu, then choose New and New Project. The following dialog will appear (note that this dialog can change a little bit depending on what add-on your have installed):
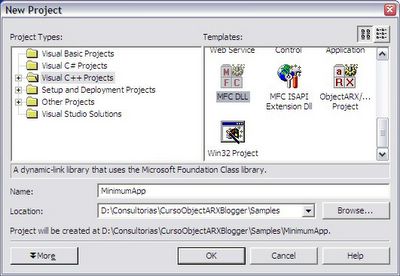
On this dialog, choose Visual C++ Projects tree node and, on the right portion select MFC DLL template. After that, specify the name and location you would like to use for this project. Click OK to continue.
After click OK the following dialog will be displayed. This dialog has two steps (in this case). The first step, called Overview, just confirm what you have entered before.
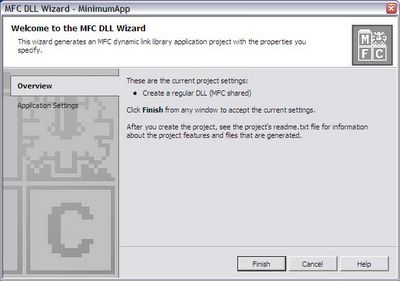
Clicking on the Application Settings step, the following page will appear inside this dialog:
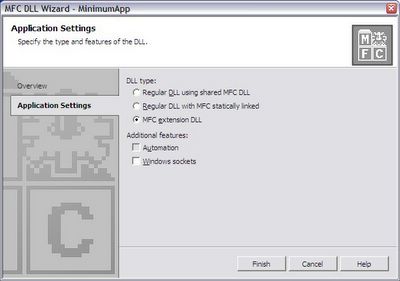
Now we will choose the MFC extension DLL that is the most adequate DLL type to build ObjectARX applications. Note that the use of MFC is not an obligation. You can build ObjectARX applications without MFC but I strongly recommend you to always use MFC because if you don't need MFC now you probably will need it in a near future.
I would like to avoid more technical discussions on this subject because this is not our focus on this course. MFC is a huge and rich library that will avoid several lines of code and will make your application safe and easy to manage. Click Finish to continue.
After clicking Finish Visual C++ will create the MFC DLL project files for you with basic implementation of some features. Remember, this is just a minimum application and we will only make a few things to turn it ready to compile, build and load into AutoCAD.
The Visual C++ environment is very intuitive and I guess you will not face much trouble to learn how to use it. Basically it has a project management area (default placed at left side), an editor area (placed at the right portion) and the command / monitor area which is placed below. The project management area uses a tab dialog bar to provide tools like Solution Explorer, Resource View and Class View among many others.
Select the Solution Explorer tab and you will see your project files, organized using a tree. This explorer can handle multiple projects but only one can be the default which has its name in bold font.
Now we will need to change some basic settings on our Visual Studio environment to allow us to compile and link ObjectARX application. As I said on previous classes, to build the application, which is a DLL, we will need to compile using the provided ObjectARX headers (.H files) and to link with ObjectARX libraries (.LIB files). The easiest way to do this is to change the global Options of Visual Studio. This will affect all projects and you won't need to do this again for new projects.
Open the Tools menu and select Options (the last entry). The following dialog will appear. Select Projects and then VC++ Directories. On the Show directories for field, select Include files. Below will appear a list of the include directories that Visual Studio already has and we will add our ObjectARX inc path which contains the desired .H files. Click on the folder icon and click on the ellipsis button and search for the inc path (in my case, it is placed at C:\ObjectARX 2004\inc).
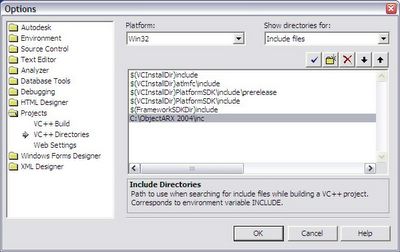
Don't click OK. Now we need to add the library files directory. Select Library files on the Show directories for field. Repeat the above procedure to add a path but this time you will add the lib path (in my case, C:\ObjectARX 2004\lib). Click OK to finish.
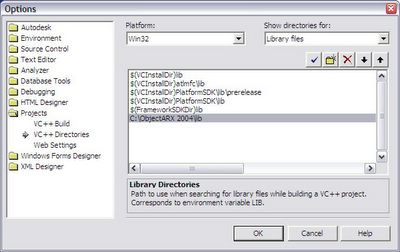
Now we need to configure our project. This will require some project settings change and some code typing. First, we will change the project settings. Right click the project name inside Solution Explorer and select Properties. The following dialog will appear. Select, on the Configuration field, All Configurations. This will allow us to change both Debug* and Release* settings at the same time.
On the Configuration Properties node, select C/C++ and Code Generation. The right portion of this dialog will display a list with several properties. Select the Runtime Library entry and chance its value to Multi-threaded DLL. This is a requirement to make our DLL compatible with AutoCAD environment.
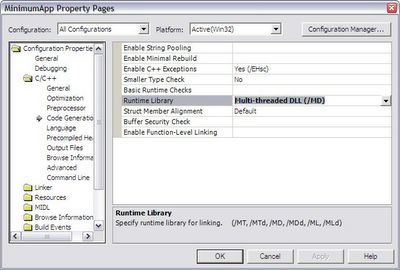
Now, select the Linker node and General. On the Output File entry change the extension name from DLL to ARX. Note that Visual Studio use several macros (those names with a $ at beginning) to allow easy and flexible path configuration.
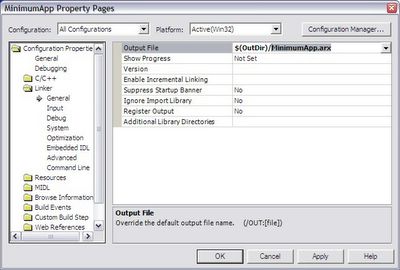
Still inside Linker node, select Input. Here we will add those libraries our application will use. This will depend on what features you are using inside your ObjectARX application. In this case, we will add just the basic two libraries called rxapi.lib and acdb16.lib.
Select the Additional Dependencies entry and click on ellipsis button. Type the two previously mentioned files. These libraries are located at lib folder. Remember that on previous classes I have talked about the features each library has built in.
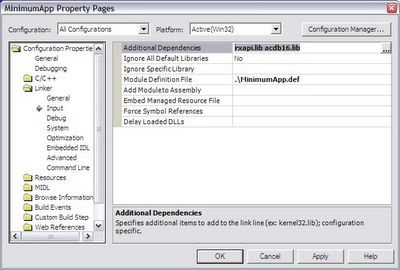
Click OK to close Project Properties dialog. Now we still need to do some code typing. The first step is to edit the DEF file which is placed at Source Files folder of your project at Solution Explorer. Double click the DEF file and it will appear at the right portion of Visual Studio window. We will need to add the following lines, under the EXPORTS section of this file:
acrxEntryPoint PRIVATE
acrxGetApiVersion PRIVATE
Pay attention to the name between quotes in front of LIBRARY section inside this file. This name must be the same name you have entered on the output file. In other words, if your project generates a ABCD.arx file you need to have LIBRARY "ABCD" inside the DEF file.
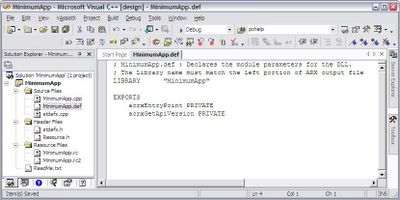
The next step is to change our StdAfx.h file which is the key compilation file. We will need to inform Visual Studio to use Release version of MFC libraries when our project is being compiled using the DEBUG directive.
To do that, open the StdAfx.h file which is located at Header Files folder inside Solution Explorer. Before the #includeline, insert the following code:
After click OK the following dialog will be displayed. This dialog has two steps (in this case). The first step, called Overview, just confirm what you have entered before.
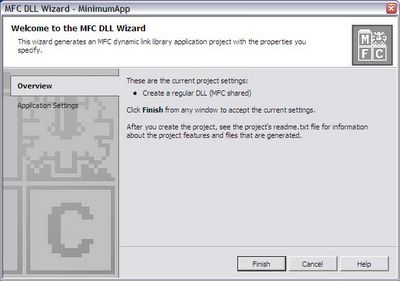
Clicking on the Application Settings step, the following page will appear inside this dialog:
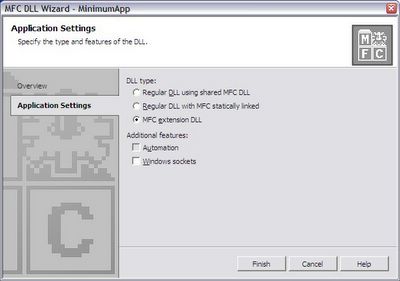
Now we will choose the MFC extension DLL that is the most adequate DLL type to build ObjectARX applications. Note that the use of MFC is not an obligation. You can build ObjectARX applications without MFC but I strongly recommend you to always use MFC because if you don't need MFC now you probably will need it in a near future.
I would like to avoid more technical discussions on this subject because this is not our focus on this course. MFC is a huge and rich library that will avoid several lines of code and will make your application safe and easy to manage. Click Finish to continue.
After clicking Finish Visual C++ will create the MFC DLL project files for you with basic implementation of some features. Remember, this is just a minimum application and we will only make a few things to turn it ready to compile, build and load into AutoCAD.
The Visual C++ environment is very intuitive and I guess you will not face much trouble to learn how to use it. Basically it has a project management area (default placed at left side), an editor area (placed at the right portion) and the command / monitor area which is placed below. The project management area uses a tab dialog bar to provide tools like Solution Explorer, Resource View and Class View among many others.
Select the Solution Explorer tab and you will see your project files, organized using a tree. This explorer can handle multiple projects but only one can be the default which has its name in bold font.
Now we will need to change some basic settings on our Visual Studio environment to allow us to compile and link ObjectARX application. As I said on previous classes, to build the application, which is a DLL, we will need to compile using the provided ObjectARX headers (.H files) and to link with ObjectARX libraries (.LIB files). The easiest way to do this is to change the global Options of Visual Studio. This will affect all projects and you won't need to do this again for new projects.
Open the Tools menu and select Options (the last entry). The following dialog will appear. Select Projects and then VC++ Directories. On the Show directories for field, select Include files. Below will appear a list of the include directories that Visual Studio already has and we will add our ObjectARX inc path which contains the desired .H files. Click on the folder icon and click on the ellipsis button and search for the inc path (in my case, it is placed at C:\ObjectARX 2004\inc).
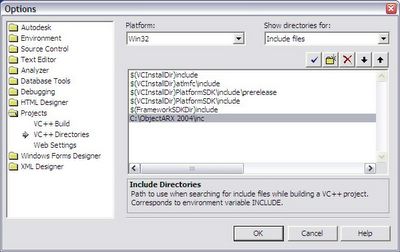
Don't click OK. Now we need to add the library files directory. Select Library files on the Show directories for field. Repeat the above procedure to add a path but this time you will add the lib path (in my case, C:\ObjectARX 2004\lib). Click OK to finish.
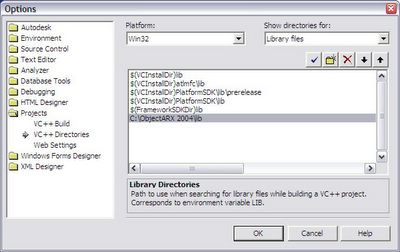
Now we need to configure our project. This will require some project settings change and some code typing. First, we will change the project settings. Right click the project name inside Solution Explorer and select Properties. The following dialog will appear. Select, on the Configuration field, All Configurations. This will allow us to change both Debug* and Release* settings at the same time.
On the Configuration Properties node, select C/C++ and Code Generation. The right portion of this dialog will display a list with several properties. Select the Runtime Library entry and chance its value to Multi-threaded DLL. This is a requirement to make our DLL compatible with AutoCAD environment.
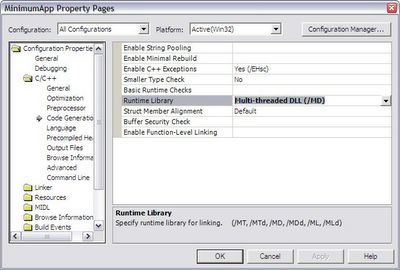
Now, select the Linker node and General. On the Output File entry change the extension name from DLL to ARX. Note that Visual Studio use several macros (those names with a $ at beginning) to allow easy and flexible path configuration.
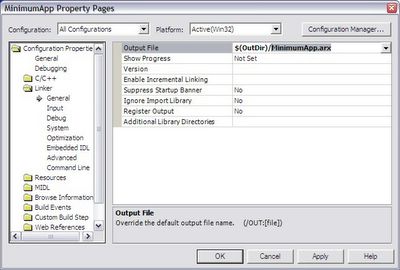
Still inside Linker node, select Input. Here we will add those libraries our application will use. This will depend on what features you are using inside your ObjectARX application. In this case, we will add just the basic two libraries called rxapi.lib and acdb16.lib.
Select the Additional Dependencies entry and click on ellipsis button. Type the two previously mentioned files. These libraries are located at lib folder. Remember that on previous classes I have talked about the features each library has built in.
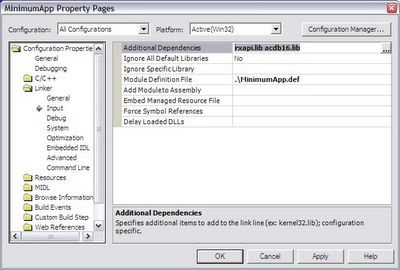
Click OK to close Project Properties dialog. Now we still need to do some code typing. The first step is to edit the DEF file which is placed at Source Files folder of your project at Solution Explorer. Double click the DEF file and it will appear at the right portion of Visual Studio window. We will need to add the following lines, under the EXPORTS section of this file:
acrxEntryPoint PRIVATE
acrxGetApiVersion PRIVATE
Pay attention to the name between quotes in front of LIBRARY section inside this file. This name must be the same name you have entered on the output file. In other words, if your project generates a ABCD.arx file you need to have LIBRARY "ABCD" inside the DEF file.
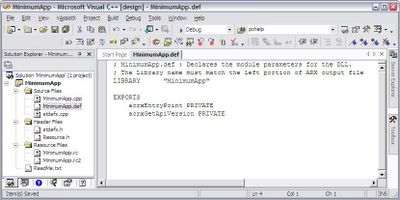
The next step is to change our StdAfx.h file which is the key compilation file. We will need to inform Visual Studio to use Release version of MFC libraries when our project is being compiled using the DEBUG directive.
To do that, open the StdAfx.h file which is located at Header Files folder inside Solution Explorer. Before the #include
#if defined(_DEBUG) && !defined(_FULLDEBUG_)
#define _DEBUG_WAS_DEFINED
#undef _DEBUG
#pragma
message (" Compiling MFC header files in release mode.")
#endif
Now, scroll to the end of this file and add the following lines to manage the _DEBUG symbol back and to include basic .H files our application will need:
#ifdef _DEBUG_WAS_DEFINED
#define _DEBUG
#undef _DEBUG_WAS_DEFINED
#endif
// ObjectARX Includes
#include "rxregsvc.h"
#include "acutads.h"
The last step is to add our acrxEntryPoint method which is our application start point. Open the CPP file of your application which has the same name that you have set to your project plus the CPP extension. It is placed inside Source Files folder. Open if and scroll down to the end. Add the following lines:
// ObjectARX ENTRYPOINT
extern "C" AcRx::AppRetCode acrxEntryPoint(AcRx::AppMsgCode msg, void* appId){switch(msg) {return AcRx::kRetOK;
case AcRx::kInitAppMsg:
acrxUnlockApplication(appId);
acrxRegisterAppMDIAware(appId);
acutPrintf(_T("\nMinimum ObjectARX application loaded!"));
break;
case AcRx::kUnloadAppMsg:
acutPrintf(_T("\nMinimum ObjectARX application
unloaded!"));
break;
}
}
Now we are ready to build our application. Open Build menu and select Build Solution (or F7 key). Visual Studio will compile and link and build your project. If you have followed all above steps carefully it will generate the application without any error.
Start AutoCAD and run APPLOAD command which will show the following dialog. Browse to your project and you will find the application inside the Debug folder which is the default compilation type. Select it and click Load button. A message will appear at the bottom of this dialog telling you that your ARX was successfully loaded or not!
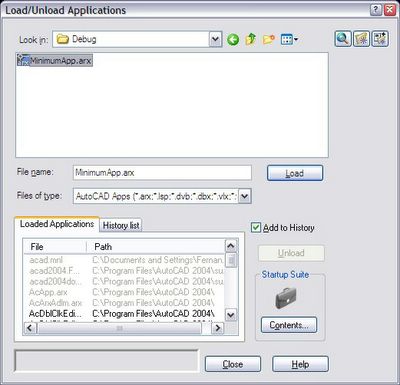
That's it, your first ObjectARX application is loaded and is running inside AutoCAD!
Next class we will do the same using the ARXWizard. Stay tuned!
49 comments :
Since you're just getting started on this...
Why not use ARX.NET instead? It's a lot easier to setup - the "Minimum application" is almost trivial.
Hello,
I'm using .NET
Class 3a cover the minimum application from scracth without using the ARXWizard.
Class 3b, that is almost ready to go, will cover the same minimum application but using ARXWizard.
My intention is to show what ARXWizard does and not leave users blind.
Wizards are great but you should always know what they do for you because if they fail (and trust me they will) you will need to fix the code by yourself.
Hope you are enjoying this course.
See you next class.
Not Visual Studio .NET, but the .NET APIs in AutoCAD.
With the .NET APIs, your "Minimal application" becomes almost trivial and you don't have to deal with a bunch of ObjectARX infastructure.
I think everyone should first try any new ARX development using the .NET APIs.
Hi Daniel,
Yes, you are correct but there are two reasons that I will not cover .NET API on this course:
1.It is not currently finished and it allows only you to use part of AutoCAD API because it is not fully exposed (Autodesk will have to work hard to expose the whole API);
2.I will cover custom entities and objects topic on this course. This feature is only possible through ObjectARX.
Thank you.
hmm...as i know, objectARX applications can not be compiled under VC7.1 due to incompatible between VS2002 and 2003.
my biggest issue is i have already updated to vs.net 2003 so i cant even use this can i???
Hello,
You will need to request a downgrade from Microsoft. As far as I know they will charge you only for the media.
Regards,
Fernando
tried this and build failed. using Visual Studio 2002 - SP1 with the Object ARX 2006 package from Autodesk.
failure appears to have nothing to do with ARX. Probably something to do with default settings becuase the build fails on a include file called new.h. Here is the build log.
<<<
------ Build started: Project: arx_minimum_app_01, Configuration: Debug Win32 ------
Compiling...
stdafx.cpp
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(76) : error C2448: 'message' : function-style initializer appears to be a function definition
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(84) : error C2143: syntax error : missing ';' before '__cdecl'
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(84) : error C2065: 'new_handler' : undeclared identifier
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(84) : warning C4229: anachronism used : modifiers on data are ignored
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(84) : error C2501: 'set_new_handler' : missing storage-class or type specifiers
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(84) : error C2143: syntax error : missing ';' before 'throw'
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(86) : error C2039: 'new_handler' : is not a member of 'std'
c:\Program Files\Microsoft Visual Studio .NET\Vc7\include\new.h(86) : error C2873: 'new_handler' : symbol cannot be used in a using-declaration
Build log was saved at "file://c:\Documents and Settings\Bill\My Documents\Visual Studio Projects\arx_minimum_app_01\Debug\BuildLog.htm"
arx_minimum_app_01 - 7 error(s), 1 warning(s)
---------------------- Done ----------------------
Build: 0 succeeded, 1 failed, 0 skipped
>>>
Hello,
ObjectARX 2004,2005 and 2006 does not support VS2002 SP1.
If you just get the sample project and compile it should work fine with VS2002 SP0 (initial release).
If you have installed the VS2002 SP1 by yourself, give a try and uninstall it.
If this not solve your problem you really need to check your ObjectARX SDK files and, as a last effort, reinstall VS2002 SP0.
Hope this help.
Regards,
Fernando.
Hi,
About the build error:
In file stdafx.h, you need to put in a single line the command:
#pragma message("...")
This sends a message to the Output window while compiling.
Nice work, Fernando!!
João
Hi
I have a similar problem as anonymous user before me.
You said:
"ObjectARX 2004,2005 and 2006 does not support VS2002 SP1."
What about OARX 2007 and VS2005?
Hi,
Regarding to VS2002 SP1 it is not officially supported by Autodesk so you need to use VS2002 SP0.
On AutoCAD 2007, you need to use VS2005. Today I have no information about VS2005 Service Packs and respective compatibility with ObjectARX 2007.
Please check ObjectARX 2007 documentation for further information.
Regards,
Fernando.
Hi Rebekh,
VS2005 can be used only with ObjectARX 2007 and AutoCAD 2007.
To be compatible with AutoCAD 2004,2005 or 2006 you need to use VS2002 and ObjectARX 2004. Note that are some differences among ObjectARX 2004,2005 and 2006 but if you use only features existing on 2004 it will run fine inside 2005 and 2006.
So if you plan to be compatible with all 2004,2005 and 2006 I would recommend you to compile with ObjectARX 2004.
Regards,
Fernando.
Hi,
on building the project I got the fatal error message: use the /MD switch
So I changed the Project Properties by Right clicking the project name inside
Solution Explorer and selecting Properties, C/C++ Folder, Commandline.
In the field additional Options I added /MD. After this the project was successfully built.
With kind regards
Frank
I'm using vb.net to create autoCAD applications. Is that method works for vb.net as well or only for C++.net? Thanks for help in advance.
regards Jarek
Hi jaroslaw,
This Blog is only about ObjectARX C++. There is a great Blog about .NET programming:
http://through-the-interface.typepad.com/
Regards.
Hi..
This site is cool...
Just what I wanted to learn.
But I've got a problem from the beginning...
Using AutoCad 2007, ObjectARX 2007, VS 8.0..
I followed and built as you said..
And got 114 errors..
Can't write all of them so there're just a few...
And please understand the error messages would be wierd.. 'cuz i'm using my language edition NOT an ENGLISH one.. so I translated..
Please help me!!
<<<
------ Build started: Project: arx_minimum_app_01, Configuration: Debug Win32 ------
Compiling...
stdafx.cpp
c:\program files\microsoft visual studio 8\vc\include\sal.h(226) : error C2448: 'message' : function-style initializer appears to be a function definition
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(530) : error C2146: syntax error : missing ';' before 'time_t'
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1710) : error C2146: syntax error : missing ';' before 'locinfo'
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1710) : error C4430: missing format specifier. assuming int......
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1710) : error C4430: missing format specifier. assuming int......
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1711) : error C2146: syntax error : missing ';' before 'mbcinfo'
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1711) : error C4430: missing format specifier. assuming int......
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1711) : error C4430: missing format specifier. assuming int......
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1729) : error C2146: syntax error : missing ';' before 'lc_id'
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1729) : error C4430: missing format specifier. assuming int......
c:\program files\microsoft visual studio 8\vc\include\crtdefs.h(1729) : error C4430: missing format specifier. assuming int......
c:\program files\microsoft visual studio 8\vc\include\new.h(60) : error C2143: syntax error : missing ';' before '__cdecl'
c:\program files\microsoft visual studio 8\vc\include\new.h(60) : error C2065: 'new_handler' : undeclared identifier
c:\program files\microsoft visual studio 8\vc\include\new.h(60) : error C2146: syntax error : missing ';' before '_NewHandler'
(((((omission))))))
MinimumApp - 114 error(s), 24 warning(s)
---------------------- Done ----------------------
Build: 0 succeeded, 1 failed, 0 skipped
>>>
Hi June,
Have you tried to compile one of the samples that come with ObjectARX2007?
From the error list seems to be some syntax error into your files.
Could you send me your project by e-mail?
Regards,
Sent e-mail to fpmalard@yahoo.com.br with a .zip file (nealy 5M)..
Thanks~
Have a good day~~~^^
Thank you for your mail.
Really appreciate that.
There aren't over 100 errors any more..
But.. got another error.
LINK : fatal error LNK1104: 'rxapi.lib' can not be opened
Sorry to bother you.
June,
Check if your *.lib folder is correct at Visual Studio Options (like the sample shows).
Further, check if this folder has some kind of access restrictions.
Regards.
Oh~~ terribly sorry..
I made a mistake..
There's a problem on setting.
(it took so looooong time to figure it out..)
Thank you!!
Hello Fernando,
I am a beginner in C++, I just took a course in it this semester and as a final project I have to do something like this: given a cross section in Autocad, computing it's moment of inertia and then showing the closest standard section in Excel. In class we just work on console projects and we don't work with Visual C++.
I was using your blog as a tutorial to get familiar with objectARX till this class that I read that we have to change the global Options of Visual Studio and this is something to do with Visual C++. So I don't know if your blog still good for me or not. Could you please give me a hint about that, I am totally clueless.
Thanks
Sara
Hi Sara,
You need to study VC++ and MFC. These are the tools you will need to create complex ObjectARX applications.
Take your time and study these things before getting deep into ObjectARX.
Remember that ObjectARX is recommended only for high performance and custom entity type applications.
If you application is simple I would recommend the C# language.
Best Regards,
I use the latest vesion of ObjectARX which is the 2008 release. Can we use VS2002, ObjectARX_2008, Autocad_2008. What is the support mix for having the functionality working and to ensure backward compatibility.
Hello,
No, you can´t. ObjectARX 2008 requires VS2005. If you plan to keep your product compatible with both families (2204,2005 and 2006) and (2007,2008 and 2009) you will need to use two project files. The first family can be compiled with VS2002.
Note that 2007, 2008 and 2009 require your application to be UNICODE compatible.
Of course if you use some specific feature from 2007 it will not compile with previous releases. In this case you can use #ifdef compiler switch to create custom compilations specific to each family.
Best regards,
I downloaded VC++ 2005 Express edition, I have Autocad Electrical 2008 and downloaded ObjectARC 2008, but there is something still missing :((
1. I don't know why the ARX wizard fails to install. It keeps telling me that I've to have VS 2005 installed. I wonder do I have to get the full licensed VS version or can I still use the Express edition version?
2. Can I use VS 2008 express, or must it be the 2005 one?
3. I ignored the wizard and tried to follow your instructions on how to create a project from scratch but I am having hard time creating an MFC dll project. Again I am not sure if this is an express edition problem or I am just doing something wrong?
4. IF I can the express edition, is there any limitations to what I can do with it?
Thanks a lot in advance for your help
Hi Mohammed,
I'm not aware of all VC++ 2005 Express edition but one is that it does not came with a Resource Editor so you need to create Resource script files (.rc) by yourself. This is really a pain.
Further it does not include both MFC and ATL. Probably it has some limitations regarding third party plug-ins installations like ARXWizard and this explain the problem you are experiencing.
I strongly recommend you to acquire at least the Professional version of VC++ 2005 to create your applications.
Regards.
Hi Fernando,
I got the same errors as June.K. 114 erros 24 warnings and the same set of errors mentioned in the comment.
How did you fix this code?
Thanks
Mohammed
Hi all, Im using AutoCAD 2007, ObjectARX 2007 and Visual Studio 2005 Version 8.0 and .NET Version 2.0.
Everything worked fine after I fixed 3 things:
- In StdAfx.h, the line: "message (" Compiling MFC header files in release mode.")" has a line feed / return, be sure it is all in one line deleting the return char. (Thanks Joao)
- Same problem in the .cpp file, the lines beginning with "acutPrintf" both had line feed /returns. Be sure they are in one line.
- Finally in ObjectARX 2007 the lib "acdb16.lib" does not exist, use "acdb17.lib" instead.
Hope this helps.
Great Job Fernando, Thanks!!!
Claudio
Hello Fernando!
I got crazy with these menus!
I have a simple menu file trying to load at AutoCAD 2007/8 start-up:
As I understand from ObjectARX documentation, there are 2 ways to deal with menus: one using “menuload ” / “cuiload” or “_menuload ” / “_cuiload” (it seems to work identically) or using COM following the example from Polysamp sample.
I try both methods and I would like to ask you some questions about them:
1.Using menu/cuiload
I have tried to load a file menu in two ways: using an old mnu file format or using a cui file resulted as a conversion of Autocad of the same .mnu file. The loading I try to invoke from both situations: in kInitAppMsg and kLoadDwgMsg using the following code:
Adesk::Boolean bIsLoaded=acedIsMenuGroupLoaded(_T("MYMENU"));
if(!bIsLoaded)
acedCommand(RTSTR, _T("_menuload"), RTSTR,_T("MyMenuFile.cui"),RTNONE);
When the arx file is automatically loaded by the "acad.rx" I got no menu , nor toolbar loaded!
Loading the arx file through "appload" command I can get only the toolbar but no menu!
I do not understand what is the problem, becose when I manually load the original mnu file using cuiload command and after the conversion of the file, the menu+toolbar are loaded!, but programatically I can not succeed!
2.Using COM
Using COM is ok, but I do not know how to set some bitmaps for menu items! I have found no documentation in ObjecArx to show how to do this!
Any idea ?
Ioan
Hi Ioan,
1. I suspect the problem is due the acedCommand() call from inside initialization events. At this startup stage the interface may not be available yet.
Try to fire the command using ads_queueexpr(). If you are using 2008, you need to declare it as:
extern "C" void ads_queueexpr( ACHAR *);
It will execute the command next time AutoCAD is quiescent.
2.I think the Menu image feature is not exposed through COM interface.
Regards,
Thank you Fernando!
Using ads_queueexpr is much better , but I still can not see the menu. The toolbar is present.
For menu, the mnu file look like:
***MENUGROUP=MYMENU
***POP8
ID_1 [MM]
ID_1_1 [CMD1]^C^C^Pcmd1
ID_1_2 [CMD2]^C^C^Pcmd2
...
I do not understand what is the problem, is a simple menu.
Anyway a step forward!
Best regards!
Ioan
Hello,
I'm using Microsoft Visual Studio C++ 6.0, following your steps in this lecture. Up until the point:
"Now we need to configure our project..."
I managed to do on 6 versions successfully as well, however after this I'm getting lost. First - I don't get "Configuration" dialog when I "Project -> properties" - I only get a small window, called "Project Folder Properties" where I can't write or change anything, so I don't know how to change both Debug* and Release* settings.
In order to get to C/C++ Code Generation I go through right click Project->Settings andchange to "Multithreaded DLL".
On the same way I reach to "Link" - change "Output File name" extention to .arx and store the 2 required libraries in
Link->Input->Object/Library Modules
I do the required changes in .def file, stdafx.h and adding a new cpp file where are store the main code - as you've described.
However, when I want to build arx_project.arx I get the following error:
rxapi.lib(libinit.obj) : fatal error LNK1103: debugging information corrupt; recompile module
Can you please explain me what does it mean and what should I do.
Many thanks in advance!!
Boris
Boris,
You need to Right click the project's name node inside Solution Explorer tree and then select Properties.
You are accessing the project's properties via "Properties" tab which is not the same thing.
The errors you have described seem to be a consequence of no project configuration done.
Regards.
Hello Fernando Thank your great blog here.
I was following this steps from net
in the Configuration properties\C++\General\Additional Include Directories insert the paths to the include folders, which are \inc and \inc-win32
in the Configuration properties\Linker\General\Additional Library Directories insert the paths to the library folder, which is \lib-win32
in the Configuration properties\Linker\Input\Additional Dependencies insert the following libraries: rxapi.lib acdb18.lib acge18.lib acad.lib acedapi.lib
If you’re planning to go 64-bit, replace “win32″ with “x64″ in the folders’ names. Now, your project should build without any problems and generate a .ARX file.
But I havent fixed this error and gonna crazy.
my configuration is
vista home premium 64 bit autocad 2010 or 2011 64 bit
Error 1 fatal error C1083: Cannot open include file: ‘arxHeaders.h’: No such file or directory ***
Barta
Hi Barta,
I prefer to configure my Visual Studio (under Tools |Options|Project and Solutions|VC++ Directions).
There you need to add your SDK paths to Include Files, Library Files and also Reference files (in this case use the same path used for Include Files).
Inside each specific project you don't need to explicitly specify those LIB files you are linking because the Visual Studio linker is smart enough to search into the Library path and bring in only those libraries it needs. In some special cases you would need to explicitly specify a library but I think this is not your case.
Once you do that every new project won't need to be configured again. Of course if you move this project to another machine it will need to have its Visual Studio configured the same way.
Good luck!
I was having the same problem as JuneK.
after a long search and compare, it turns out the problem is in this line, it should be:
#pragma message (" Compiling MFC header files in release mode.")
(in the same line)
Hello,
#pragma is a compiler instruction and it shouldn't give any errors if correctly used.
Have you checked your VS path configuration and if you have the proper ObjectARX release installed?
Regards.
Hello,
I'm using autocad 2012 x64, ObjectARX 2012 and visual studio 2010. I succesfully compiled the minimum application. However when I load it in autocad I get a message: 'autocad is attempting to load ..arx, which is not compatible with autocad'. But when I select 'Load this application' it just loads without a problem. Any idea? Thanks in advance.
marc,
Did you install VS2010 service pack?
http://www.microsoft.com/en-us/download/details.aspx?id=23691
It is required to build compatible ARX modules.
I think this will solve your problem but please let me know about it.
Regards,
I installed servicepack 1, but nothing changed. I wanted to try the wizard, but keep getting build errors.
This is strange. Are you sure you have a valid Visual Studio install?
Things I would suggest you to try:
- Reinstall VS2010 and its SP1
- Reinstall ObjectARX 2012
- Make sure your VS2010 Settings are properly pointing to the ARX folders (note that you need to add both inc and its counterpart 32/64 inc
- Try to compile and run one of the ARX samples
There must be something wrong there...
Regards,
Hi Fernando:
I'm trying to build arx projects in visual studio 2012 (vs2012) but when I try to link rxapi.lib and acdb19.lib to my project, vs2012 does not shown the ellipsis button on Additional Dependencies. Do you know how to include these two links in vs2012?
Thanks a lot in advance.....
oscar,
The Dependencies now are per project.
- Right click your project at the Solution explorer;
- Click Properties;
- Go to Configuration Properties tree node;
- Select VC++ Directories;
- Put your paths into the 3 fields: Include, Reference (same as include) and Library;
If you have more than one project into your Solution you will need to repeat this process for all of them.
Hope it helps you.
Regards,
Hi Fernando:
I don’t know if I told you before but since you still show it, I’ll tell you this:
In the lines we ned to insert to StdAfx.h, I need to comment the line:
message (" Compiling MFC header files in release mode.")
because it gives error. I think it can help anybody that is having the same problem.
Additionally, I want to ask you about the following error:
rxapi.lib(libinit.obj) : error LNK2038: mismatch detected for 'RuntimeLibrary': value 'MD_DynamicRelease' doesn't match value 'MDd_DynamicDebug'
It is curios that the error just happens when I build the project in Debug mode. If I build the project in Release mode that error disappears.
No big deal but I am afraid it can give me problems later.
Regards and thanks for your help.
Oscar.
Hi Oscar,
It seems the Project Configuration is incorrect in regards to the /MD or /MDd configurations.
Take a look here:
http://msdn.microsoft.com/en-us/library/2kzt1wy3(v=vs.110).aspx
Hope it helps.
cant u help me? i got some error:
Error 3 error LNK2001: unresolved external symbol "__declspec(dllimport) private: static void * __cdecl AcHeapOperators::allocRawMem(unsigned __int64)" (__imp_?allocRawMem@AcHeapOperators@@CAPEAX_K@Z) F:\study\thuc tap\object ARX\arx2\arx2\arx2.obj arx2
Error 4 error LNK2001: unresolved external symbol "__declspec(dllimport) private: static void __cdecl AcHeapOperators::freeRawMem(void *)" (__imp_?freeRawMem@AcHeapOperators@@CAXPEAX@Z) F:\study\thuc tap\object ARX\arx2\arx2\arx2.obj arx2
Error 5 error LNK2001: unresolved external symbol "__declspec(dllimport) public: __cdecl AcGePoint3d::AcGePoint3d(double,double,double)" (__imp_??0AcGePoint3d@@QEAA@NNN@Z) F:\study\thuc tap\object ARX\arx2\arx2\arx2.obj arx2
Error 6 error LNK1120: 3 unresolved externals F:\study\thuc tap\object ARX\arx2\x64\Release\arx2.arx arx2
Hello,
First, please do not post the same question several times. The website has a Spam protection so all posts need to be approved before they appear. Be patient.
You are having a linking problem so you are either missing the Libraries you need or pointing to incorrect Libraries according to the ObjectARX and Visual Studio versions you are using.
Check these requirements: http://arxdummies.blogspot.com.br/2005/01/requirements.html
Regards.
Post a Comment